Formik and ZOD -Validating Forms with Schema ✌️💡
This blog post takes a deep dive into the world of Formik and Zod, offering an in-depth guide on how to validate forms with schemas. Formik simplifies form state management, while Zod empowers you to define precise data constraints with ease.
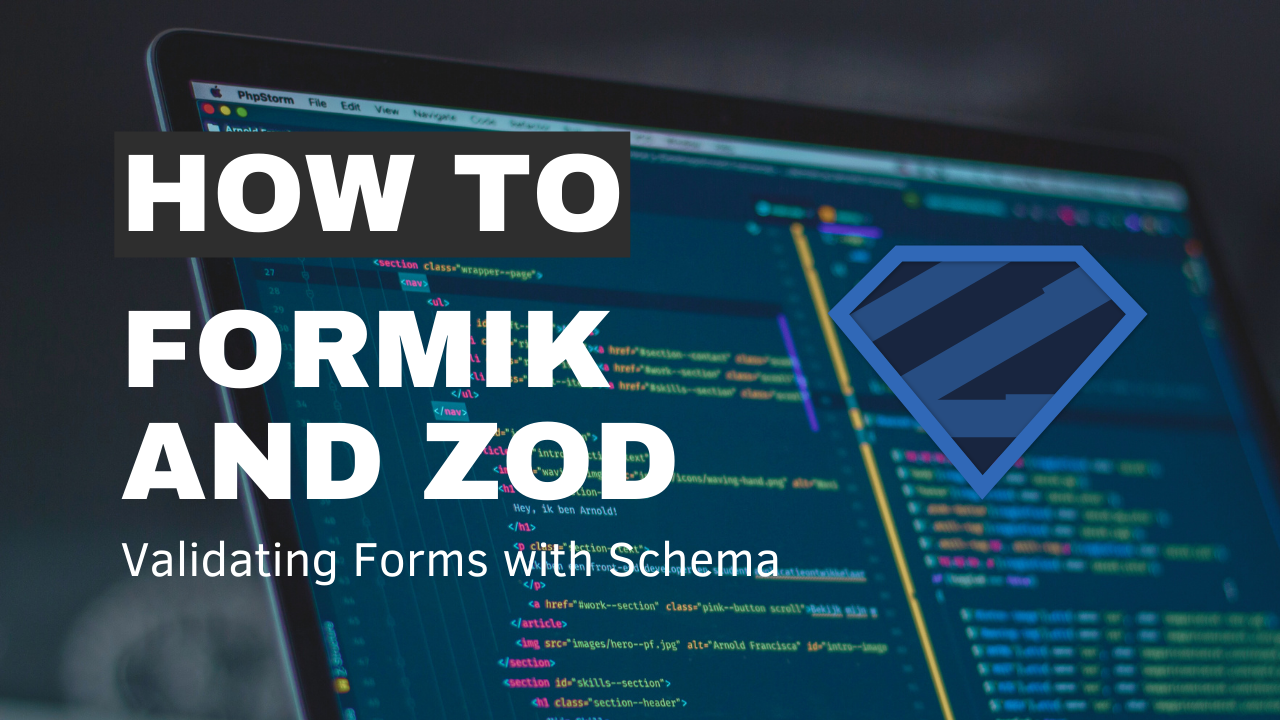
Form validation is a crucial part of creating user-friendly and error-free web applications. While libraries like Formik help streamline form management in React applications, combining it with a powerful validation library like Zod can make the process even more efficient and robust. In this blog post, we'll explore how to validate Formik forms with Zod and create a seamless user experience.
Why Formik and Zod?
Formik is a popular library for building forms in React. It simplifies form state management, handling form submissions, and managing form validation. Zod, on the other hand, is a robust and type-safe JavaScript runtime validation library. Together, they create a powerful duo for building forms that are not only easy to work with but also highly reliable and secure.
Setting up your project
To get started, make sure you have a React project set up. You can create one using create-react-app
or any other preferred method.
Install Formik and Zod using npm /yarn/pnpm :
pnpm add formik zod
# OR
npm install formik zod
Creating a Formik Form
Let's start by creating a simple Formik form. We'll create a registration form as an example:
import React from 'react';
import { Formik, Field, Form, ErrorMessage } from 'formik';
const RegistrationForm = () => {
return (
<Formik
initialValues={{
username: '',
email: '',
password: '',
}}
onSubmit={(values) => {
// Handle form submission
console.log(values);
}}
>
<Form>
<div>
<label htmlFor="username">Username</label>
<Field type="text" id="username" name="username" />
<ErrorMessage name="username" component="div" />
</div>
<div>
<label htmlFor="email">Email</label>
<Field type="email" id="email" name="email" />
<ErrorMessage name="email" component="div" />
</div>
<div>
<label htmlFor="password">Password</label>
<Field type="password" id="password" name="password" />
<ErrorMessage name="password" component="div" />
</div>
<button type="submit">Submit</button>
</Form>
</Formik>
);
}
export default RegistrationForm;
Adding Zod Validation
Now, let's integrate Zod for validation. First, import Zod at the top of your file:
import { z } from 'zod';
Next, define your validation schema. You can define the rules for each field using Zod. For our registration form, let's add some basic validation:
const ValidationSchema = z.object({
username: z.string().min(3, 'Username is too short').max(20, 'Username is too long'),
email: z.string().email('Invalid email address').min(5, 'Email is too short'),
password: z.string().min(8, 'Password is too short'),
});
ZOD validation schema
Now, we'll use Formik's validate
prop to perform Zod validation on the form:
import React from 'react';
import { Formik, Field, Form, ErrorMessage } from 'formik';
import { z } from 'zod';
const ValidationSchema = z.object({
username: z
.string()
.min(3, "Username is too short")
.max(20, "Username is too long"),
email: z.string().email("Invalid email address").min(5, "Email is too short"),
password: z.string().min(8, "Password is too short"),
});
type FormValues = z.infer<typeof ValidationSchema>;
const RegistrationForm = () => {
const validateForm = (values: FormValues) => {
try {
ValidationSchema.parse(values);
} catch (error) {
if (error instanceof ZodError) {
return error.formErrors.fieldErrors;
}
}
};
return (
<Formik<FormValues>
initialValues={{
username: '',
email: '',
password: '',
}}
validate={validateForm}
onSubmit={(values) => {
// Handle form submission
console.log(values);
}}
>
{/* ... rest of the form remains the same */}
</Formik>
);
}
export default RegistrationForm;
Form validation with ZOD
With this setup, your Formik form will now perform Zod validation when the user interacts with it. If any validation rules are not met, error messages will be displayed, ensuring that the user provides valid input.
Conclusion
Combining Formik and Zod for form management and validation can greatly enhance your React application's user experience. You have the power to define complex validation rules with Zod and seamlessly integrate them into your Formik forms. This ensures that your forms are not only easy to work with but also robust and secure.
By following the steps outlined in this guide, you can create forms that are both user-friendly and reliable, making for a better overall user experience in your React applications. Happy coding!
Source Code:
You can find the source code for this article at this link:
Thanks for reading ♥️♥️