#10FlutterChallenges β Part 4: Filtering List by Matching IDs π
Real challenges & real problems. Learn how to filter list by matching ID's in Flutter!
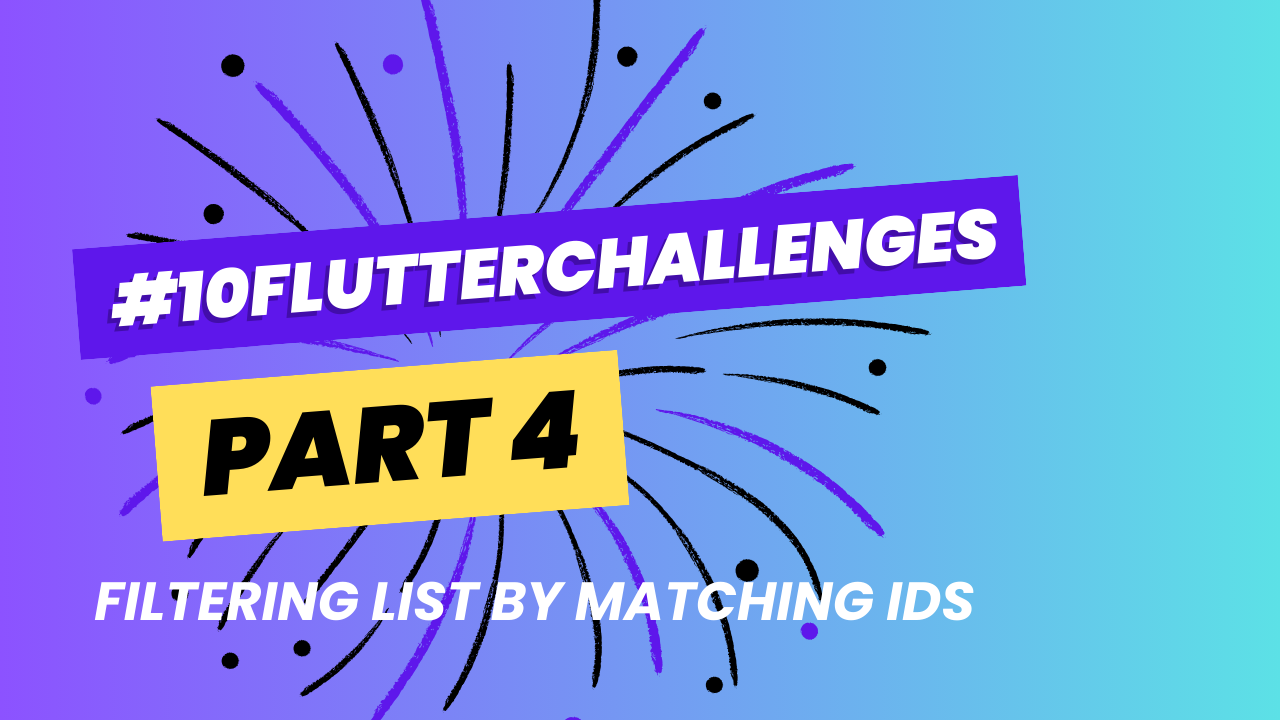
Hey there! It's been a while since the last Flutter Challenge. But we're back with this series! If you haven't seen the previous one, take a look at these links:
Part 1 β #10FlutterChallenges - Part 1: progress bar with gradient
Part 2 β #10FlutterChallenges - Part 2: switch button
Part 3 β #10FlutterChallenges - Part 3: Audio + LifeCycle service
Welcome to the Part 4 of the Flutter Challenges blog series. π
Part 4 π:
In this challenge, we will filter the list of users by their IDs. But there are a few requirements that must be met. Here they are:
- Matching IDs will be provided as a list of integers. e.g.,
final ids = [1, 3, 5]
- If the IDs are not provided, e.g
final ids = [];
β the method should return an empty list - If none of the IDs match the user ID, the method should return an empty list
- If at least one of the IDs matches the User ID, it should return only matching User's instances.
First, let's define the User
class and user's list:
class User {
int id;
String name;
User({required this.id, required this.name});
}
List<User> usersList = [
User(id: 1, name: "John"),
User(id: 2, name: "Alex"),
User(id: 3, name: "Barry"),
User(id: 4, name: "Michael"),
User(id: 5, name: "Alexandra"),
];
User class with users list
To iterate throughout this list, we will use the where method, which returns the matching element that meets the given condition:
final filteredUsers = userList.where((User user) {
print(user.id) // 1, 2, 3 ... etc
}).toList();
Iterating through user list using where method
In brackets, we have access to the instance of each individual user.
Finally, we use the toList() method, which converts the iterable returned from where method into a list of users.
The last step is to match the user IDs to the IDs from the ID's list. We can use any method for this purpose:
final idsList = [1, 3];
List<User> filteredUsers = userList.where((User user) {
return idsList.any((id) => id == user.id);
}).toList();
// [User(id: 1, name: "John"), User(id: 3, name: "Barry")]
Filtering users list by ids with where and any methods
As you can see, if the given ID's matches the user ID, the method returns the user instances.
And if there is no matching ID, the method will return an empty list like so:
final idsList = [231, 333];
List<User> filteredUsers = userList.where((User user) {
return idsList.any((id) => id == user.id);
}).toList();
// []
Filtering users list by ids with where and any methods
Also, if only partial IDs match, the method will only return matching users:
final idsList = [1, 333];
List<User> filteredUsers = userList.where((User user) {
return idsList.any((id) => id == user.id);
}).toList();
// [User(id: 1, name: "John")]
Filtering users list by ids with where and any methods
Source code
You can check out the source code from this article in this gist:
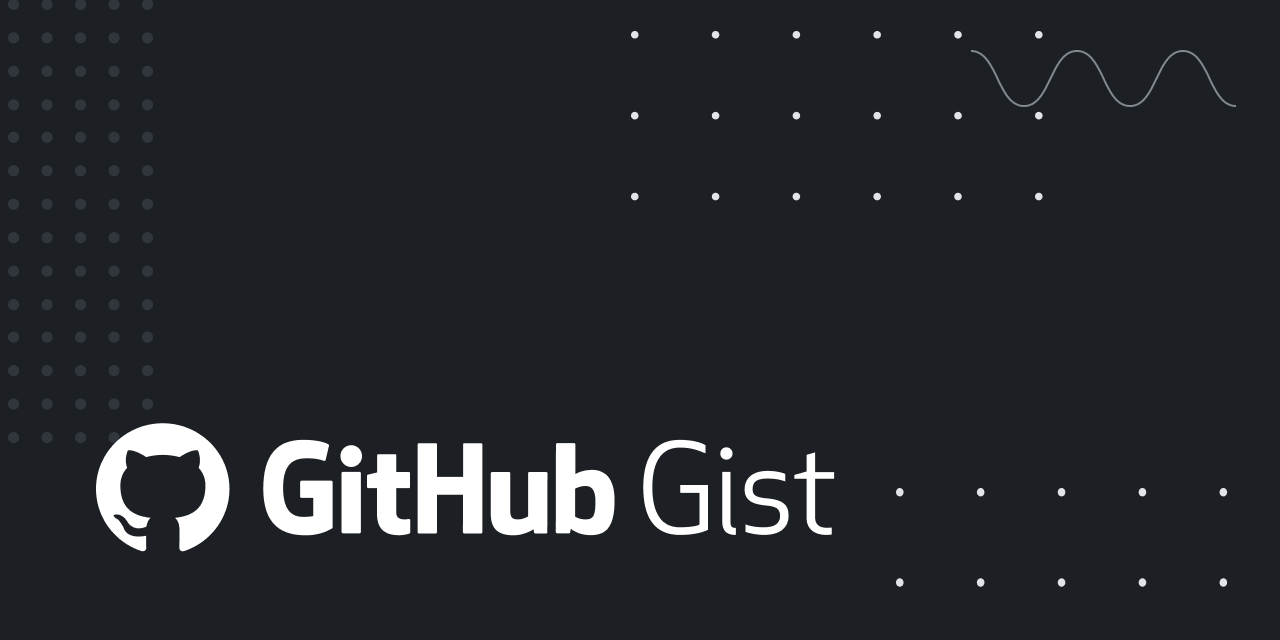
Thanks for reading β₯οΈβ₯οΈ
β¦and stay tuned! π
Also check out the rest of the Flutter Challenges:
Part 1 β #10FlutterChallenges - Part 1: progress bar with gradient
Part 2 β #10FlutterChallenges - Part 2: switch button
Part 3 β #10FlutterChallenges - Part 3: Audio + LifeCycle service